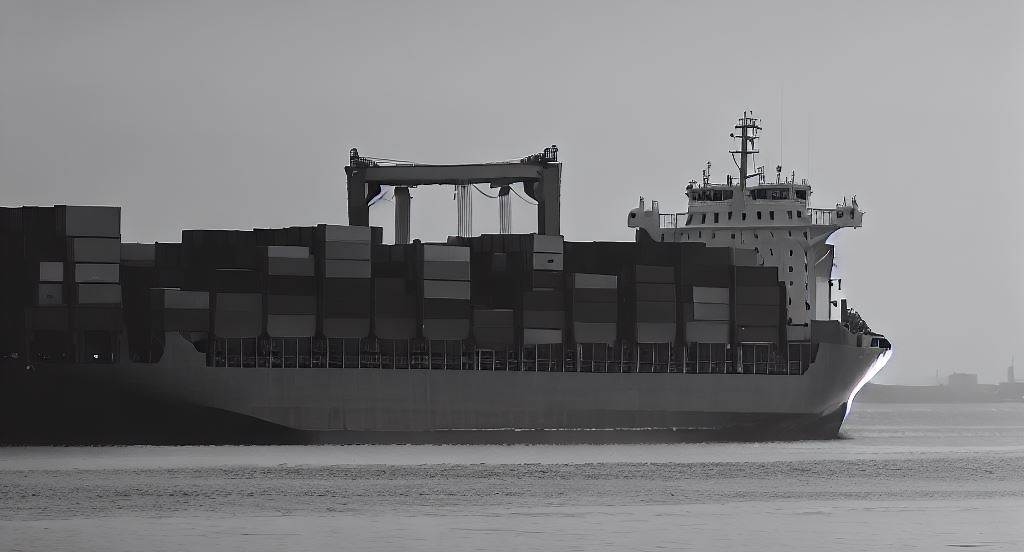
API
API to release the power of CODICI
A RESTful API is the standard way to integrate computers with each other. CODICI has also chosen to use the API to offer the power of our artificial intelligence. You use the API to ask a question, indicating what you know about your product through parameters. The API's response, in JSON format, can then be used to directly integrate into your systems.
The response from our API is in JSON format and displays five results, sorted by confidence score. The confidence score is an estimation by our artificial intelligence of how relevant the answer is. The higher the confidence score, the better the answer matches your question.
API call
Basics
The base URL for API calls is https://api.codi.ci/api/search. You authenticate yourself with a bearer token, using the key you have already received and can also find in your portal.
You can pass your search parameters in the URL using the "q" parameter to send your query to the API. An example of a request would look like this: https://api.codi.ci/api/search/?q=your_description_here
Replace "your_description_here" with the description or question you want to ask the API. Make sure to add the necessary authentication to your request, such as the bearer token mentioned earlier.
Bearer token
If you have clicked on the link, you may have noticed that you are redirected to a login page. This is because you need to authenticate yourself with a bearer token during the request. However, we have provided some examples in our GitHub to assist you with the integration. Additionally, you can also test your code against our testing environment.
Parameters
With parameters, you can specify what you are searching for a HS code. Below is an overview of all possible parameters:
q | The parameter for the product description you are searching the HS code for is "q". Please remember to URL encode the description (replace spaces with "%20" and so on). This parameter is mandatory, meaning you must include it in your API request with the encoded product description. |
Response
The response from the API is provided in the JSON format. This format is designed to be flexible, so you don't have to make changes to your integration every time a new version is released. An example of a response (to a fictional question) is shown below:
{
"data": [
{
"confidenceScore": 93,
"hs_code": "5803001000"
},
{
"confidenceScore": 75,
"hs_code": "6206300000"
},
{
"confidenceScore": 68,
"hs_code": "6302310000"
},
{
"confidenceScore": 67
"hs_code": "5304310000"
},
{
"confidenceScore": 62,
"hs_code": "5200210000"
}
]
}
If you are not familiar with the JSON format yet, it would be best to learn it online. We assume that you are already familiar with the JSON format here. JSON (JavaScript Object Notation) is a widely used data interchange format that is easy for humans to read and write, as well as for machines to parse and generate. It consists of key-value pairs and supports various data types such as strings, numbers, booleans, arrays, and nested objects.
As you can see, the response consists of an array called data. This array contains multiple collections. Each collection contains two variables. The 'hs_code' variable holds the 10-digit HS code. In the same collection, there is the 'confidenceScore' variable, indicating the AI's level of confidence in the answer. The first collection in the array is the one with the highest 'confidenceScore', which means it is considered the best advice by the AI.
Testing
To support you in implementing our API, we provide a test environment for you. The responses from this environment provide the same JSON format as the production environment but with additional fields that provide information about any issues or errors. You can access our test API at https://test-api.codi.ci/api/search. Once you have finished testing, simply remove the test- prefix from the URL, and you will be ready for production use.
If you click on the link for the test API here, you will receive a response similar to this:
{
"data": [
{
"confidenceScore": 77,
"hs_code": "0003001000"
},
{
"confidenceScore": 74,
"hs_code": "0000210000"
},
{
"confidenceScore": 73,
"hs_code": "0006300000"
},
{
"confidenceScore": 70
"hs_code": "0002334500"
},
{
"confidenceScore": 62,
"hs_code": "0002310000"
}
],
"messagecode": "1,2",
"message": "No search query was given. | No bearer token found."
}
As you can see, the response format of the test API is similar to that of the actual API, but it includes two additional fields: messagecode and message. The messagecode field contains a list of error codes separated by commas, which you can look up below. The message field provides a brief description of the same error codes, separated by the | symbol. However, please note that the content of the responses is nonsensical and will be different each time as it is solely used for testing purposes. For this reason the codes returned are in chapter 00 (first two digits of the HS-code) which does not exist.
If you don't have much experience with integrating APIs, we recommend using Postman. It is a powerful tool that allows you to extensively test APIs. Postman also supports bearer tokens through the Authorization tab by selecting "Bearer Token" as the authentication type.
Furthermore, we have provided example code in several programming languages on our GitHub repository. You can use these examples as a starting point for your integration.
If you have read through this documentation and still need assistance, please feel free to reach out to our support department at support@codi.ci. They will be happy to assist you further.
Error code overview
Below is a brief overview of the different error codes, which will be explained in detail below the table:
1 | No search query was given. | You did not provide a description in de API call. |
2 | No bearer token found. | You did not provide a bearer token. |
3 | Unknown parameters found. | You have used additional paramters that the search query does not recognize. |
4 | Client might not accept json. | The computer that did the API request did not share its ability to process JSON. |
Foutcodes
1 - No search query was given
There are two requirements when making a call to the API: the bearer token (see error code 2) and the search text. You will encounter error code 2 if you do not provide the search text. You can pass the search text using the parameter q. An example API call would look like this: https://test-api.codi.ci/api/search?q=flowered%20cotton%20dress. Note that you need to convert the search text to ensure proper handling of the API requests. This process is known as URL encoding. URL encoding adjusts characters that are not allowed in the request, enabling them to be used by the API. For example, a space is represented as (%20) and a question mark as (%3F).
2 - No bearer token found.
The bearer token is used for authentication purposes. You can find the token (a sequence of numbers and letters) in your portal. To pass the bearer token to the API, it should be included in the message header. The header should be as follows: Authorization: Bearer 1234567890
where 1234567890 is your own bearer token. You can find examples for various programming languages on our GitHub repository.
3 - Unknown parameters found.
You have used parameters in the API call that are not recognized (and therefore not listed in the overview under the section API Call). Perhaps you made a typo and intended to pass something else. The additional parameters should not cause any issues when using the API, but it is better to exclude them from the call.
4 - Client might not accept json.
The computer making a request via the API can specify the type of response it understands. The responses from our API are provided in the application/json format. The requesting computer can indicate the response it can process using the Accept header. You can include the following in the headers:Accept: application/json
Alternatively, you can indicate that any type of response will be understood by using:Accept: */*
Currently, the API always responds in the JSON format. However, to avoid potential errors in the future when modifications are made to the API, it is advisable to include the Accept header in your request. This will ensure that the response is properly handled by your application and allow for easier adaptation to future changes.
Extended testing
Once you have finished the initial integration, it is possible (for the test API only) to force specific situations to allow you to test for those as well. This is done by using the parameter cmd. There are a few possible commands that you can use:
You can combine commands by seperating them with a comma. So the command cmd=c,3 will give you 3 results with real HS-codes. Some combinations might not make sense, like cmd=u,3 which will just give you an unauthenticated error or could be vague: cmd=c,3,4 which will actually give you 4 real HS-codes because the last numeric occurence is used.
1,2,3,4 | The API will return 1, 2, 3 or 4 results respectively instead of 5. This will allow you to test what your implementation will do if the result set is smaller than 5. |
u | Force an 'Unauthenticated' error so you can test what your application does when the bearer key is incorrect or has expired. |
c | Use real HS-codes in the answers only. PLEASE NOTE: the HS-codes will be real but will have no connection to your request. They are random. |